How do single cell organisms coordinate sophisticated behaviors?
Plan
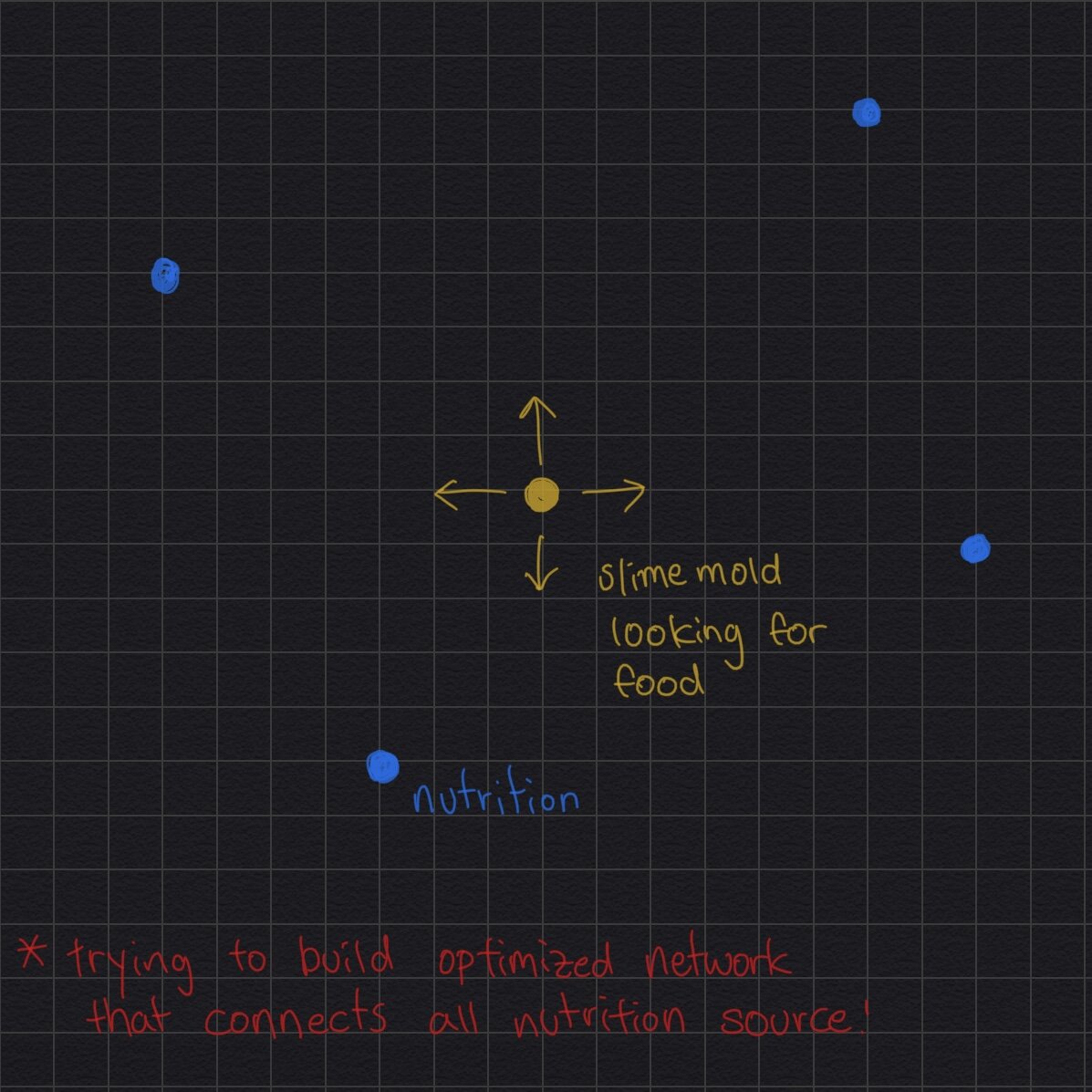
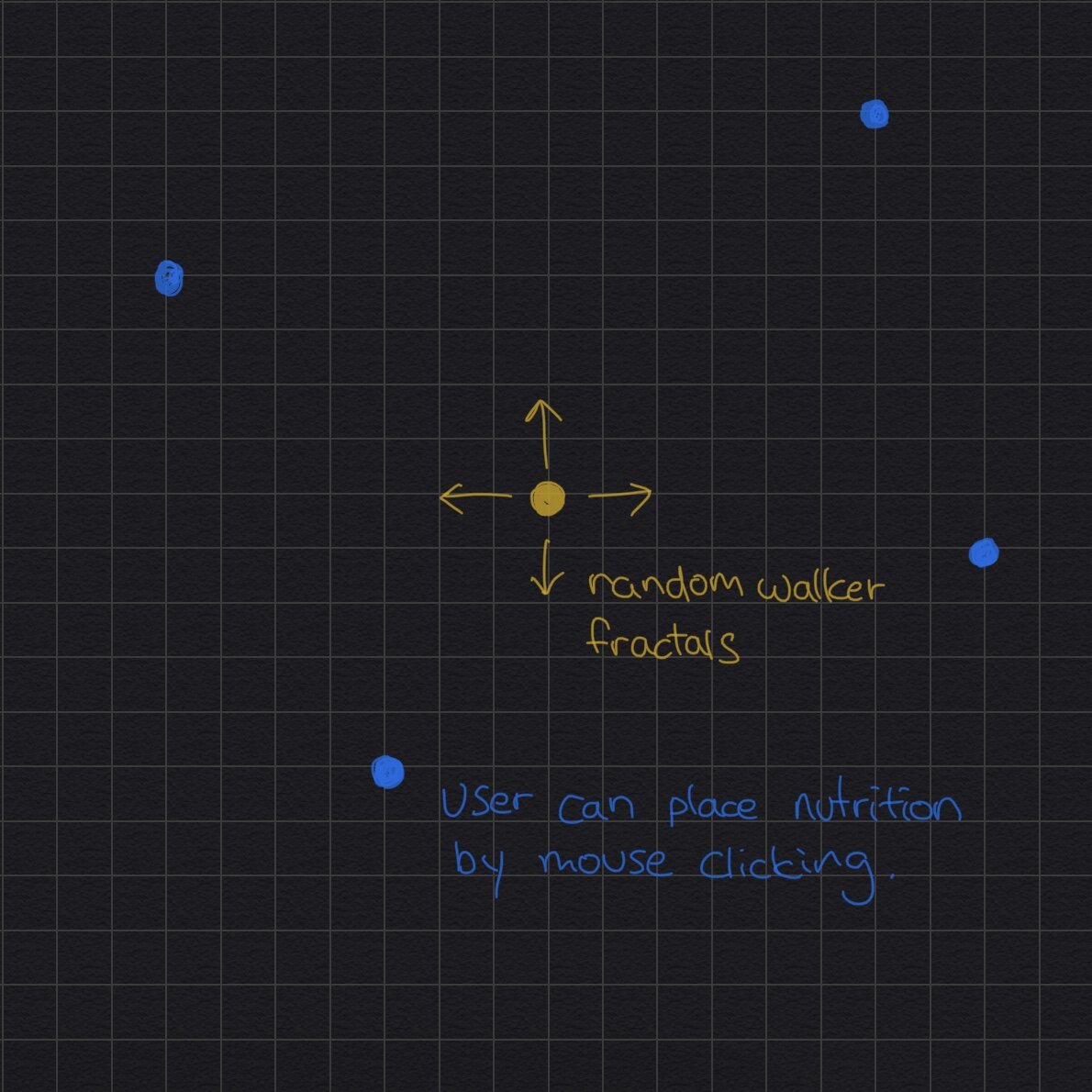
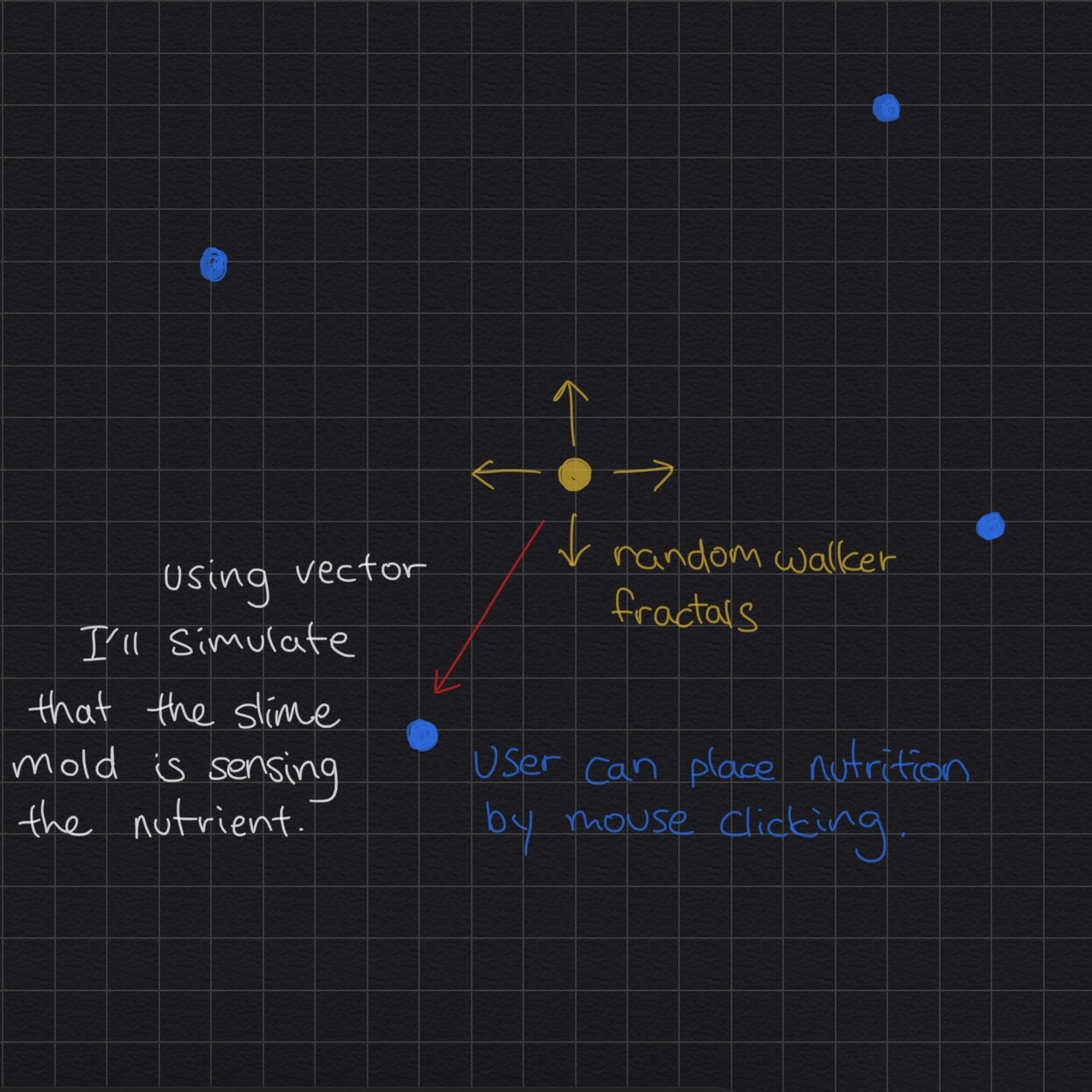

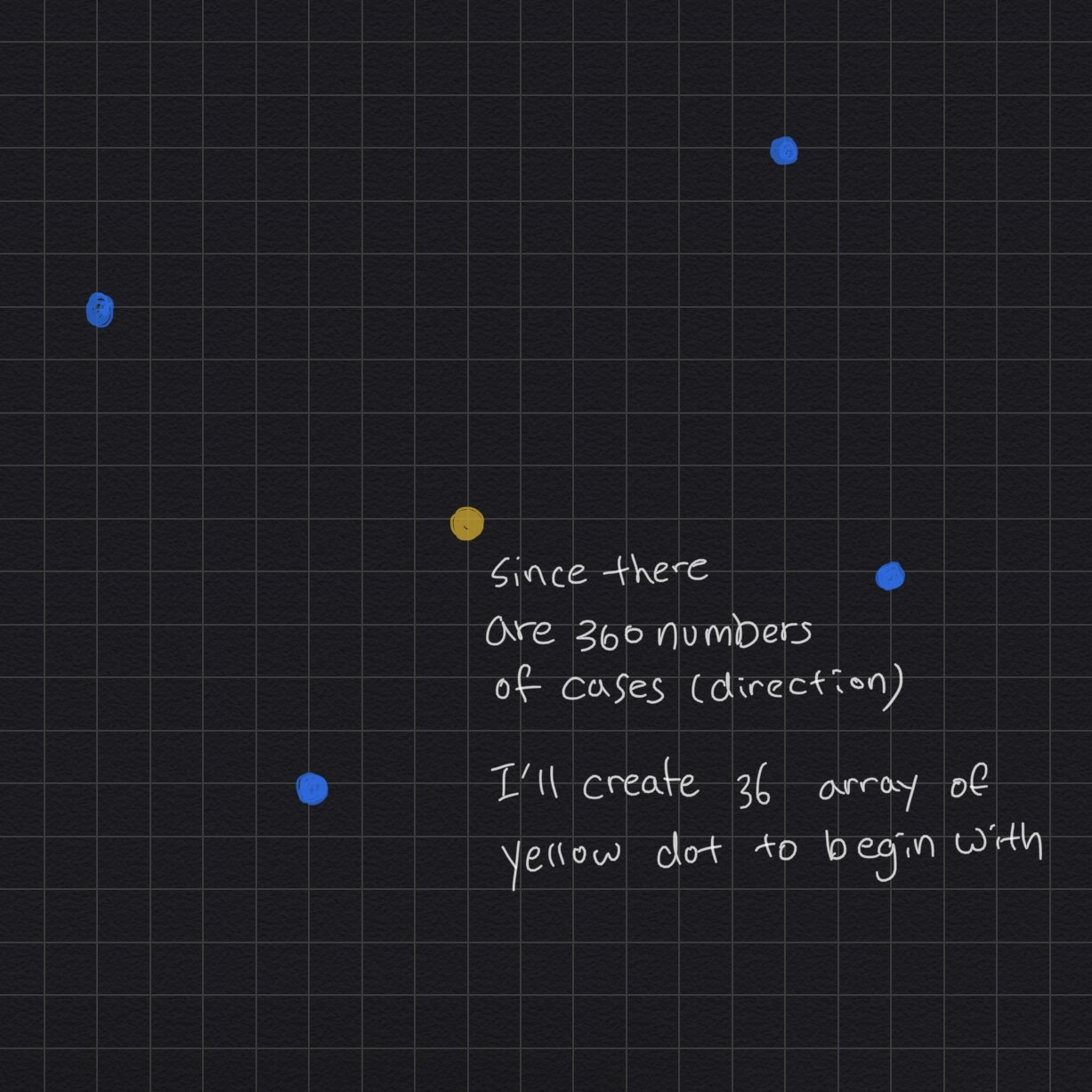
p5 - Nutrient
//user can use mouse-click to place blue dots. //yellow dot will build the optimized network connecting all blue dots //define Slime array & network array var nutrients = []; function setup() { createCanvas(800, 800); } //place Nutrient when user press mouse function mousePressed() { var n = new Nutrient(mouseX,mouseY,5); nutrients.push(n); } function draw() { background(220); // nutrients for (i=0; i<nutrients.length; i++) { nutrients[i].display(); } } //Nutrient function Nutrient (x,y,m) { //pos,vel,acc,mass (Just in case for later use) this.pos = createVector(x,y); this.vel = createVector(0,0); this.acc = createVector(0,0); this.mass = m; // color,shape of Nutrient this.display = function() { fill(0,0,255); ellipse(this.pos.x,this.pos.y,this.mass,this.mass); } }
p5 - Mold (Random Walker)
//create Array of slimes var slimes = [] function setup() { createCanvas(800, 800); background(220); for (let i = 0; i < 36; i++) { slimes[i] = new Slime(width / 2, height / 2, 0.5); } //make slimes to leave the trail for (let i = 0; i < 36; i++) { slimes[i].display(); } } function draw() { for (let i = 0; i < 36; i++) { slimes[i].display(); slimes[i].update(); } } function Slime(x, y, m) { //set up pos,vel,acc,mass this.pos = createVector(x, y); this.vel = createVector(0, 0); this.acc = createVector(0, 0); this.mass = m; //display (color, ellipse) this.display = function() { noStroke(); fill(0); ellipse(this.pos.x, this.pos.y, this.mass); } this.update = function() { this.vel.add(this.acc); this.pos.add(this.vel); this.acc.set(0, 0); //Movement of Slimes var movement = p5.Vector.random2D(); movement.mult(1.2); this.pos.add(movement); } }
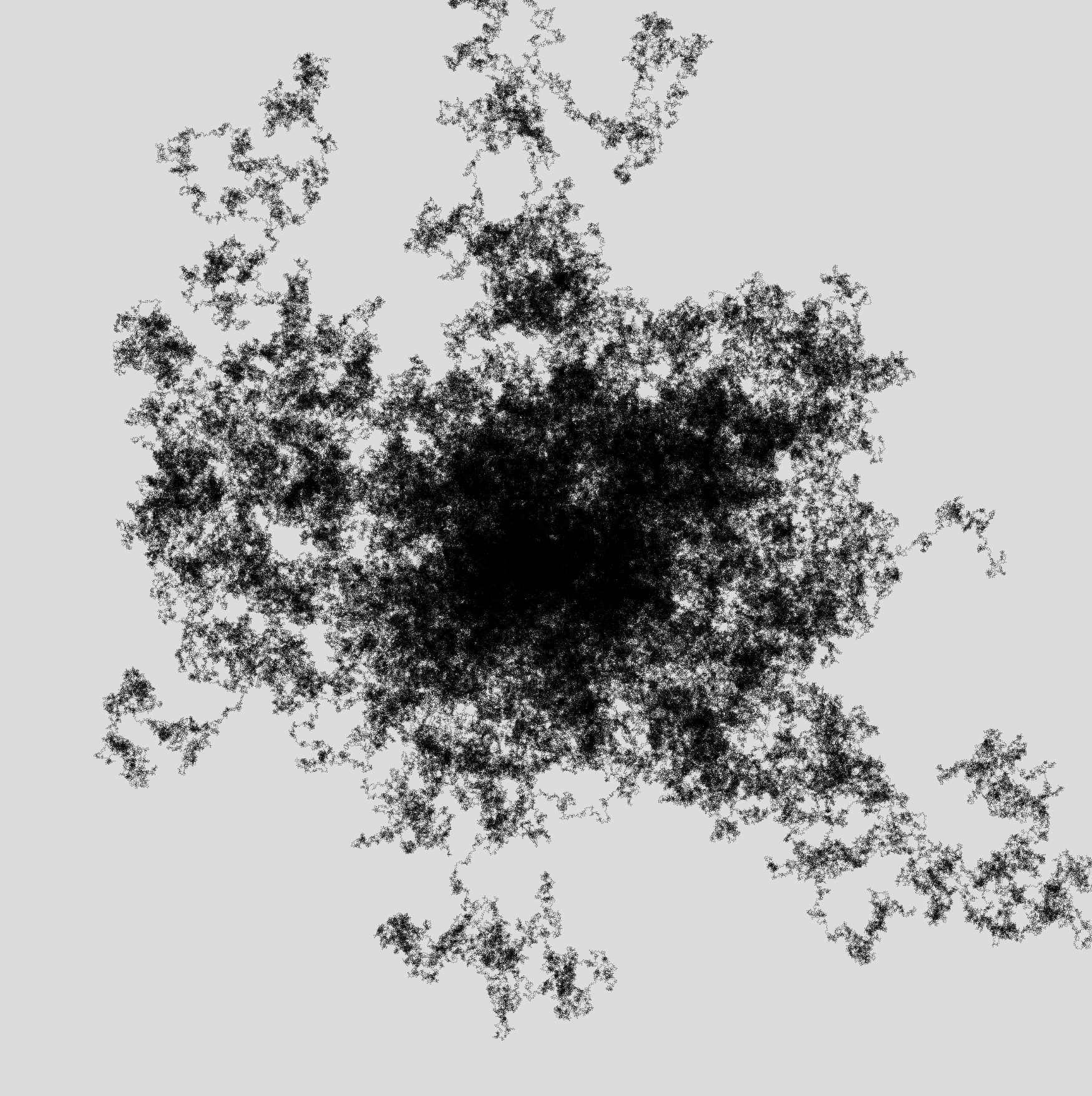
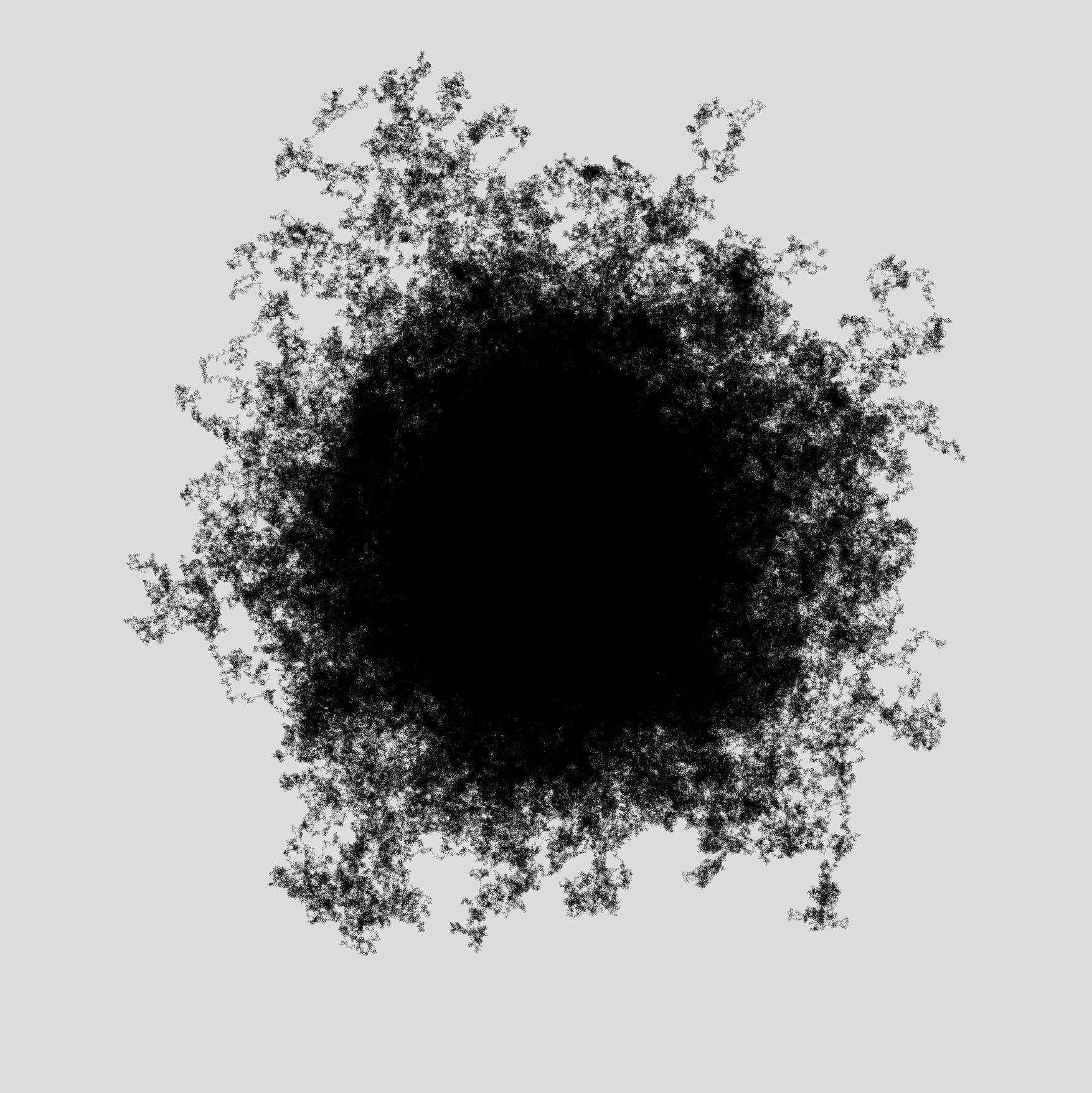
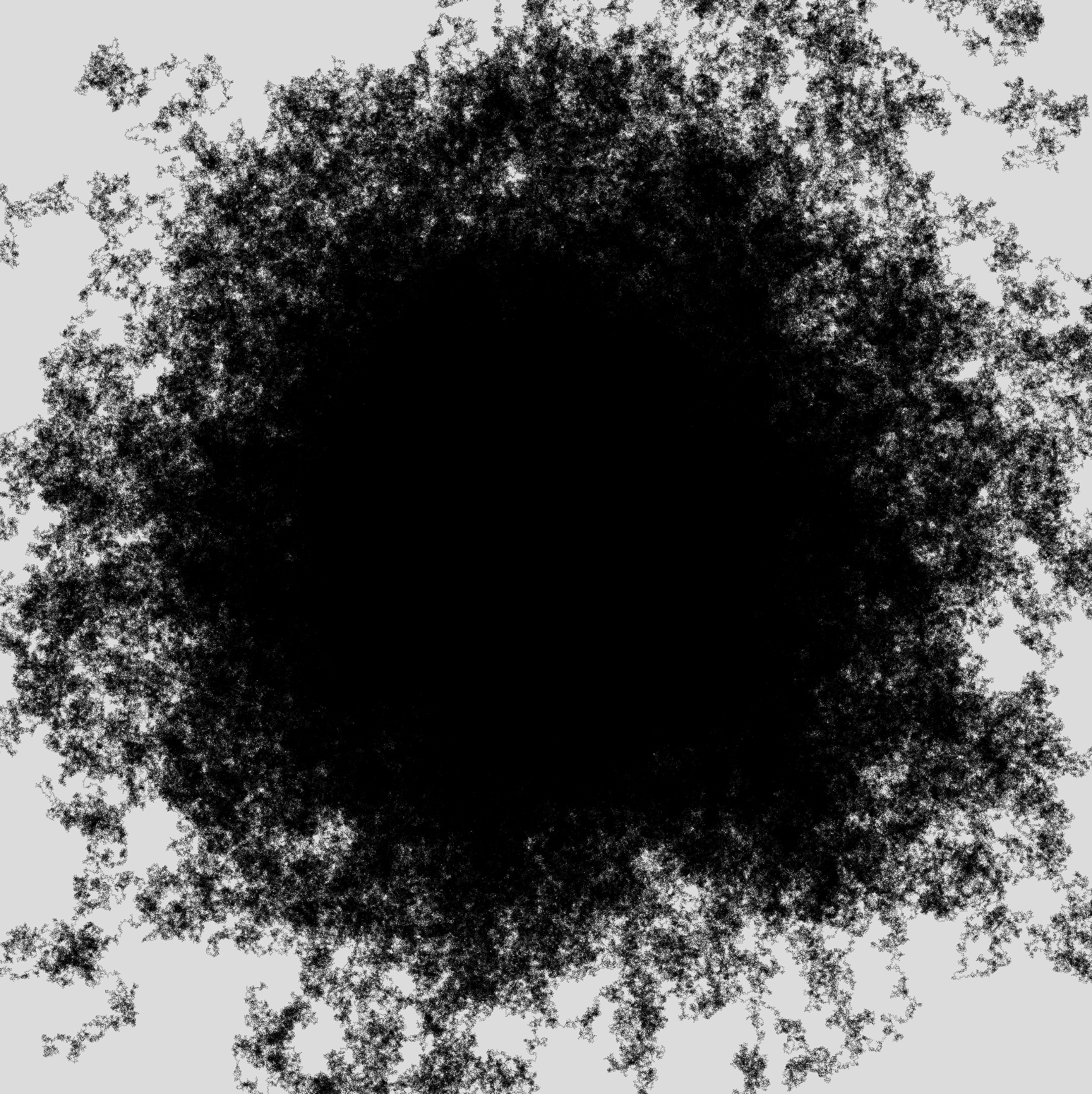