Final Project Process
Idea Sketch
Materials & Measurements
Experiments
Video
Laser Sculpturing in Space [Final project]
Inspiration
Matthew Schreiber
Reference
UTOPIA [Poetic Light]
UTOPIA
Final Video
Inspiration: James Turrell
Idea sketch & Plan-out
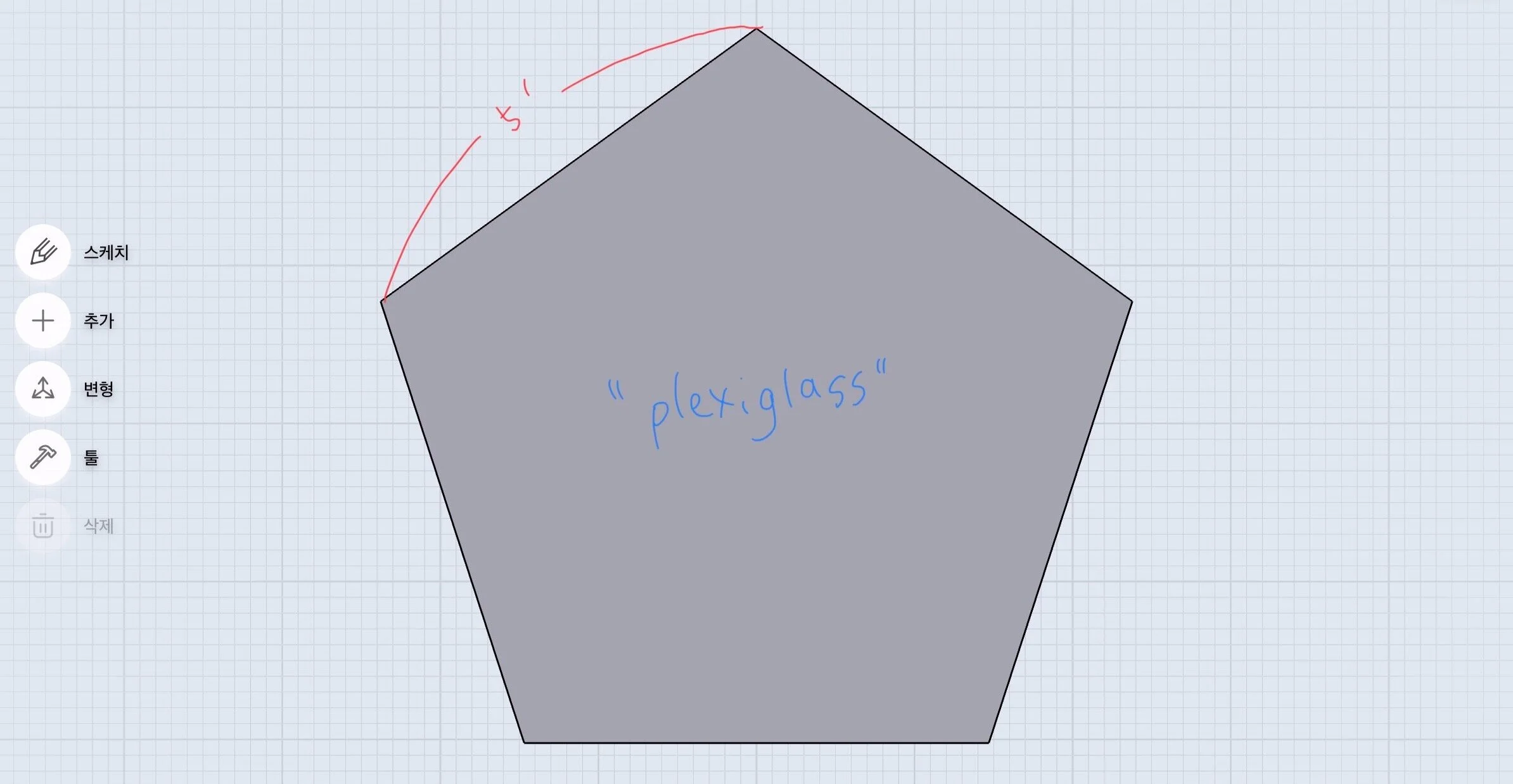
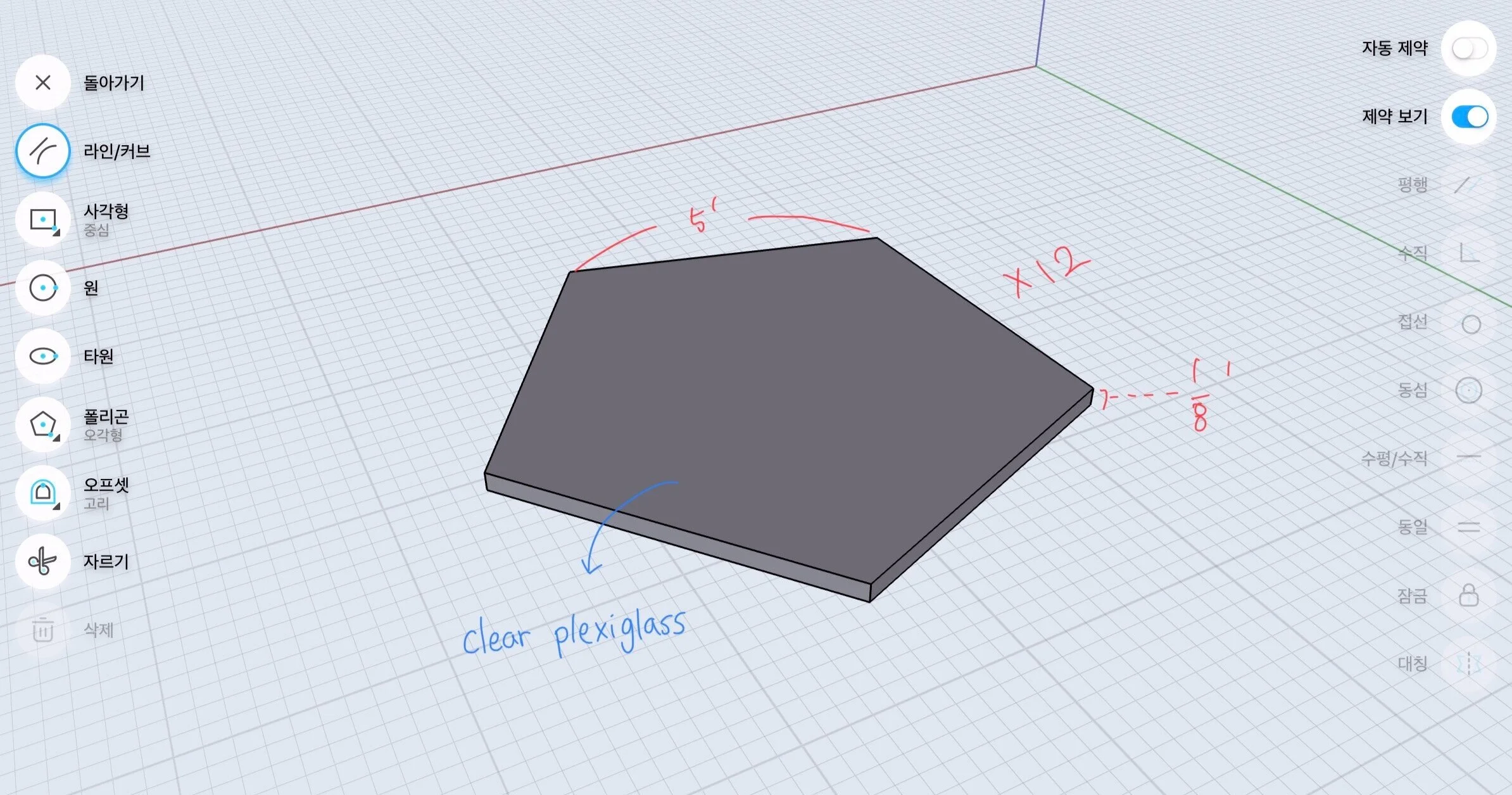

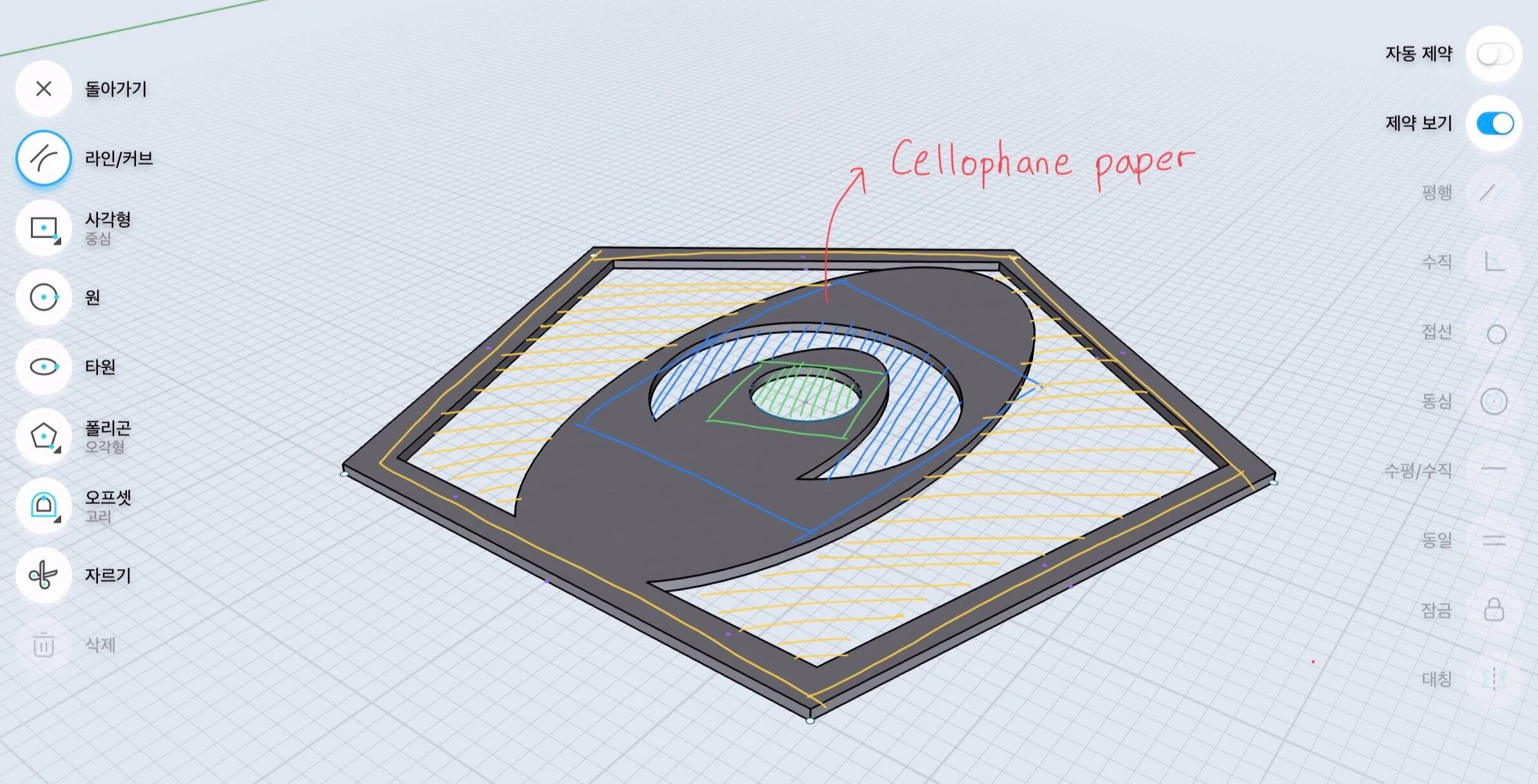

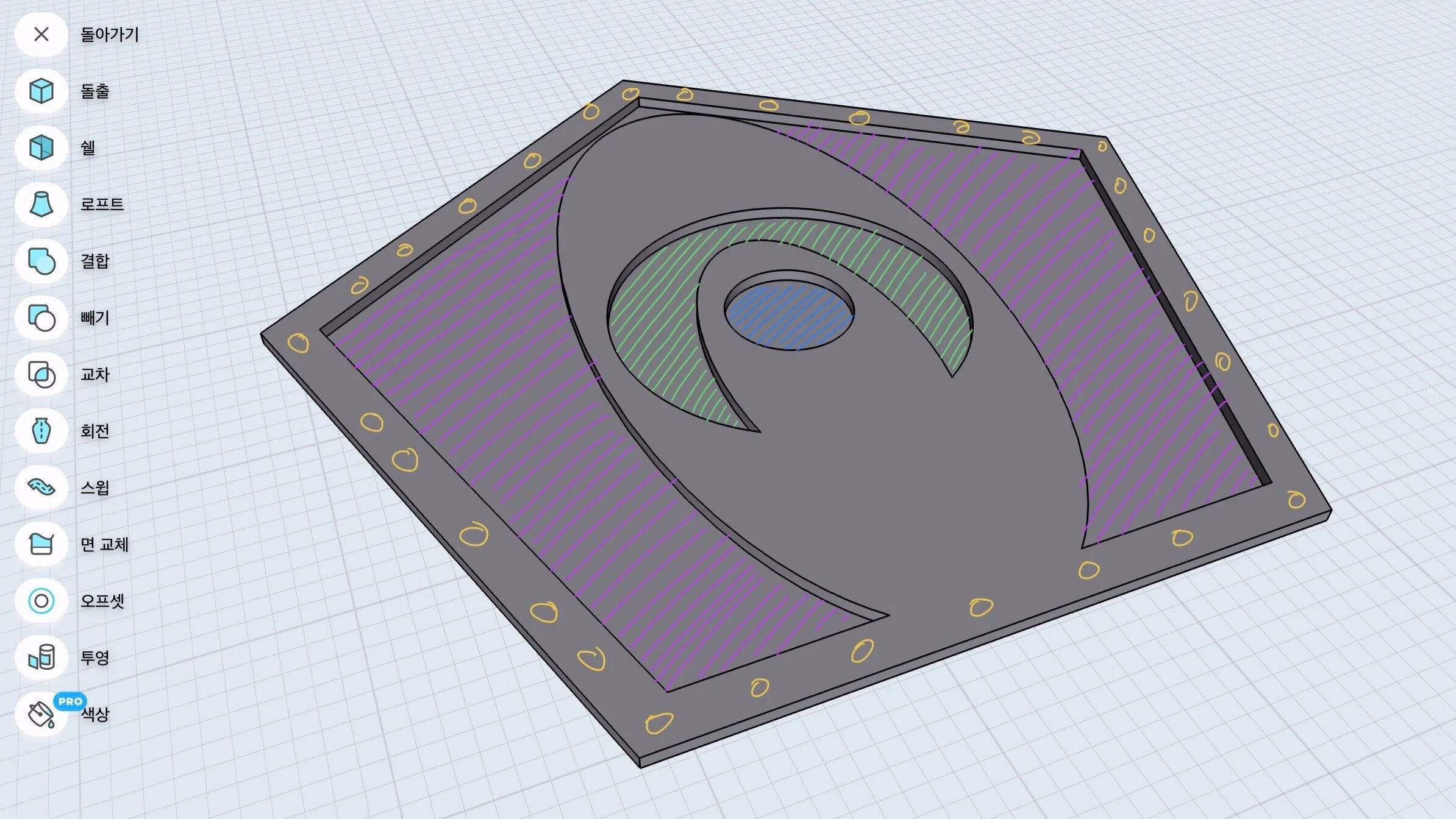
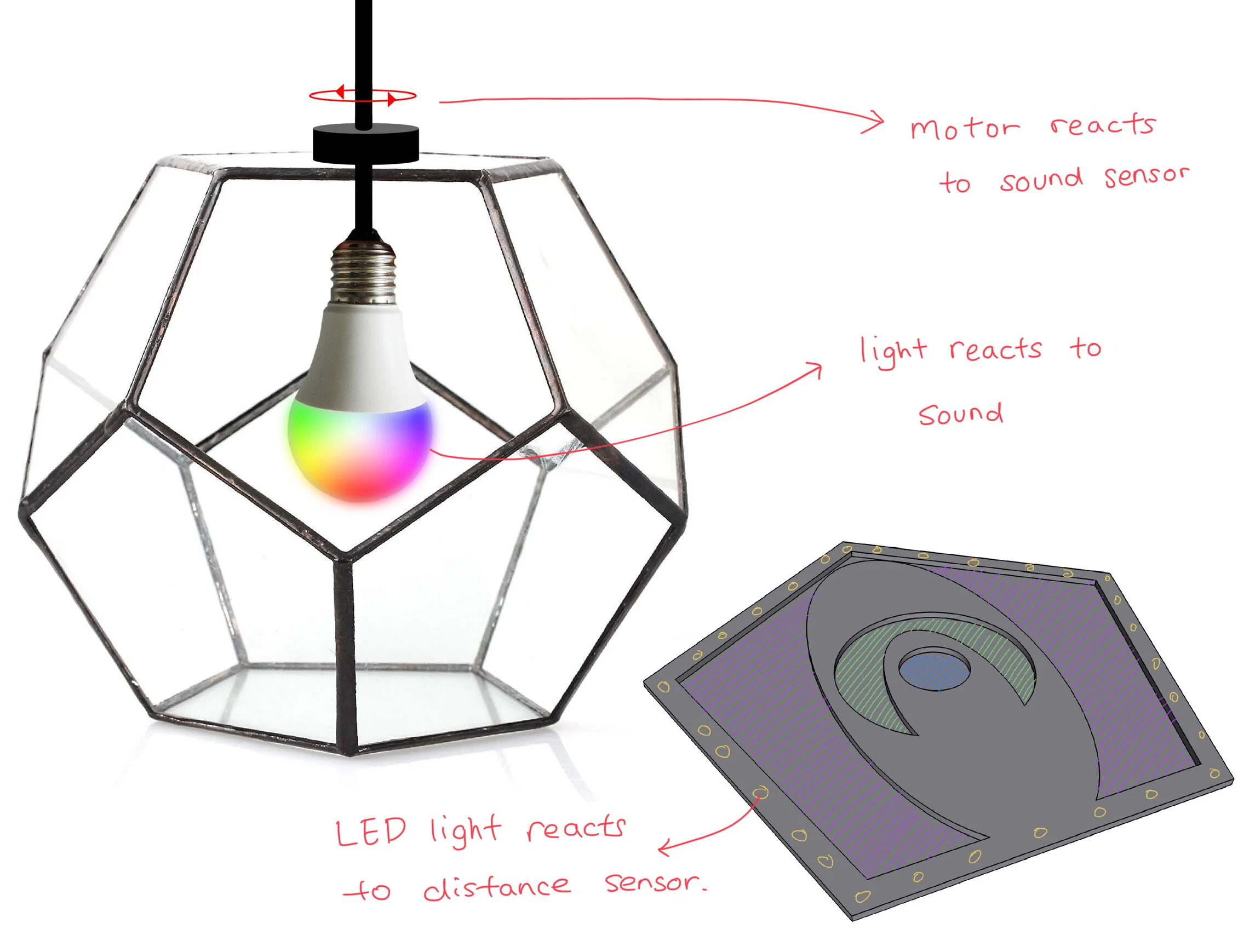
Materials: Distance sensor(Ultrasonic sensor), Sound sensor(Microphone sensor), Motor, Plexiglass, Cellophane paper, LED strip, Arduino Nano
Mockup - play around with light, shadow and shape
UTOPIA (Interactive Poetic Light)
Coding for LED strip(WS2812B) and Ultrasonic sensor
//set up variables for LED #include <FastLED.h> //import Fast.Led #define LED_PIN 5 #define NUM_LEDS 20 #define BRIGHTNESS 500 #define LED_TYPE WS2811 #define COLOR_ORDER GRB CRGB leds[NUM_LEDS]; #define UPDATES_PER_SECOND 100 CRGBPalette16 currentPalette; TBlendType currentBlending; extern CRGBPalette16 myRedWhiteBluePalette; extern const TProgmemPalette16 myRedWhiteBluePalette_p PROGMEM; //set up variables for Ultra Sonic sensor int trigPin = 11; // Trigger int echoPin = 12; // Echo long duration, cm, inches; void setup() { //setup for LED stripe Serial.begin (9600); delay( 1000 ); // power-up safety delay FastLED.addLeds<LED_TYPE, LED_PIN, COLOR_ORDER>(leds, NUM_LEDS).setCorrection( TypicalLEDStrip ); FastLED.setBrightness( BRIGHTNESS ); currentPalette = RainbowColors_p; currentBlending = LINEARBLEND; //setup for Ultrasonic Seonsor pinMode(trigPin, OUTPUT); pinMode(echoPin, INPUT); } void loop() { //animtate light //ultasonic sensor digitalWrite(trigPin, LOW); delayMicroseconds(5); digitalWrite(trigPin, HIGH); delayMicroseconds(10); digitalWrite(trigPin, LOW); pinMode(echoPin, INPUT); duration = pulseIn(echoPin, HIGH); cm = (duration/2) / 29.1; // Divide by 29.1 or multiply by 0.0343 inches = (duration/2) / 74; // Divide by 74 or multiply by 0.0135 Serial.print(inches); Serial.print("in, "); Serial.print(cm); Serial.print("cm"); Serial.println(); if (cm > 100){ ChangePalettePeriodically(); } else { static uint8_t startIndex = 0; startIndex = startIndex + 1; /* motion speed */ FillLEDsFromPaletteColors(startIndex); FastLED.show(); FastLED.delay(1000 / UPDATES_PER_SECOND); } } void FillLEDsFromPaletteColors( uint8_t colorIndex) { uint8_t brightness = 255; for( int i = 0; i < NUM_LEDS; i++) { leds[i] = ColorFromPalette( currentPalette, colorIndex, brightness, currentBlending); colorIndex += 3; } } void ChangePalettePeriodically() { uint8_t secondHand = (millis() / 1000) % 60; static uint8_t lastSecond = 99; if( lastSecond != secondHand) { lastSecond = secondHand; if( secondHand == 0) { currentPalette = RainbowColors_p; currentBlending = LINEARBLEND; } if( secondHand == 10) { currentPalette = RainbowStripeColors_p; currentBlending = NOBLEND; } if( secondHand == 15) { currentPalette = RainbowStripeColors_p; currentBlending = LINEARBLEND; } if( secondHand == 20) { SetupPurpleAndGreenPalette(); currentBlending = LINEARBLEND; } if( secondHand == 25) { SetupTotallyRandomPalette(); currentBlending = LINEARBLEND; } if( secondHand == 30) { SetupBlackAndWhiteStripedPalette(); currentBlending = NOBLEND; } if( secondHand == 35) { SetupBlackAndWhiteStripedPalette(); currentBlending = LINEARBLEND; } if( secondHand == 40) { currentPalette = CloudColors_p; currentBlending = LINEARBLEND; } if( secondHand == 45) { currentPalette = PartyColors_p; currentBlending = LINEARBLEND; } if( secondHand == 50) { currentPalette = myRedWhiteBluePalette_p; currentBlending = NOBLEND; } if( secondHand == 55) { currentPalette = myRedWhiteBluePalette_p; currentBlending = LINEARBLEND; } } } // This function fills the palette with totally random colors. void SetupTotallyRandomPalette() { for( int i = 0; i < 16; i++) { currentPalette[i] = CHSV( random8(), 255, random8()); } } void SetupBlackAndWhiteStripedPalette() { // 'black out' all 16 palette entries... fill_solid( currentPalette, 16, CRGB::Black); // and set every fourth one to white. currentPalette[0] = CRGB::White; currentPalette[4] = CRGB::White; currentPalette[8] = CRGB::White; currentPalette[12] = CRGB::White; } void SetupPurpleAndGreenPalette() { CRGB purple = CHSV( HUE_PURPLE, 255, 255); CRGB green = CHSV( HUE_GREEN, 255, 255); CRGB black = CRGB::Black; currentPalette = CRGBPalette16( green, green, black, black, purple, purple, black, black, green, green, black, black, purple, purple, black, black ); } const TProgmemPalette16 myRedWhiteBluePalette_p PROGMEM = { CRGB::Red, CRGB::Gray, // 'white' is too bright compared to red and blue CRGB::Blue, CRGB::Black, CRGB::Red, CRGB::Gray, CRGB::Blue, CRGB::Black, CRGB::Red, CRGB::Red, CRGB::Gray, CRGB::Gray, CRGB::Blue, CRGB::Blue, CRGB::Black, CRGB::Black };
Coding for Microphone sensor and DC Motor
int motorPin = 3; int sensorPin=7; boolean val =0; void setup() { pinMode(motorPin, OUTPUT); pinMode(sensorPin, INPUT); Serial.begin (9600); } void loop() { val =digitalRead(sensorPin); Serial.println (val); if (val==HIGH) { digitalWrite(motorPin, HIGH); } else { digitalWrite(motorPin, LOW); } }
Digital INPUT/OUTPUT - Tone output
//1. Range of the analog input void setup() { Serial.begin(9600); // initialize serial communications } void loop() { int analogValue = analogRead(A0); // read the analog input Serial.println(analogValue); // print it }
SOLDERING
I tried to figure out why circuit was not working. Since Arduino read and printed analog input fine, I could know there was a problem with speaker circuit. I found out that the ‘+’ wire from the speaker was not connected right. It should’ve connected to D8 not ‘+’ of a breadboard. Finally, I made a sound come off from the speaker.
//2. Play Tones void setup() { } void loop() { // get a sensor reading int sensorReading = analogRead(A0); // map the results from the sensor reading's range // to the desired pitch range: float frequency = map(sensorReading, 0, 1000, 100, 1000); // change target range will change pitch // change the pitch, play for 10 ms: tone(8, frequency, 10); }
P5 reference
Arduino Reference
Idea sketch
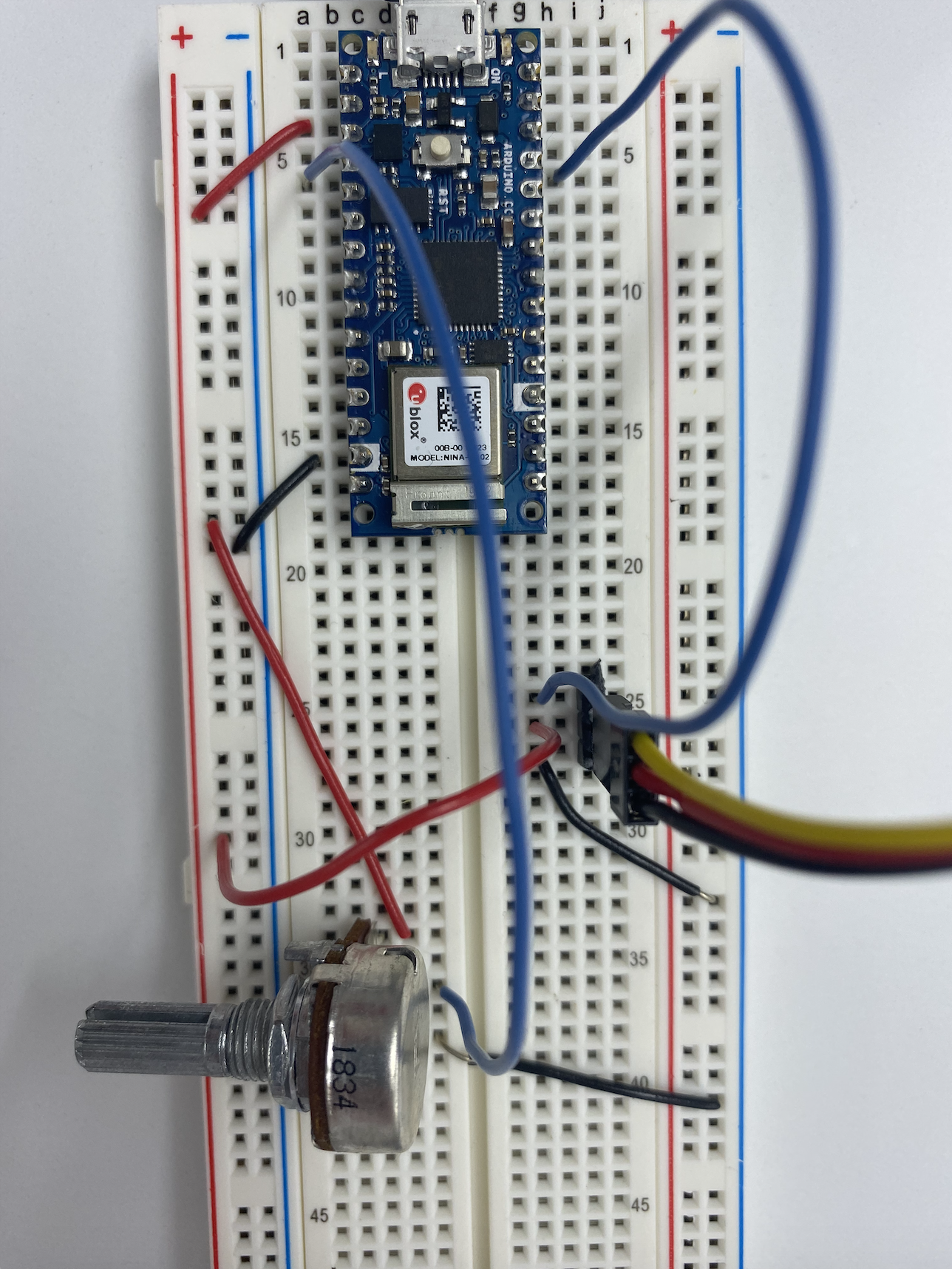
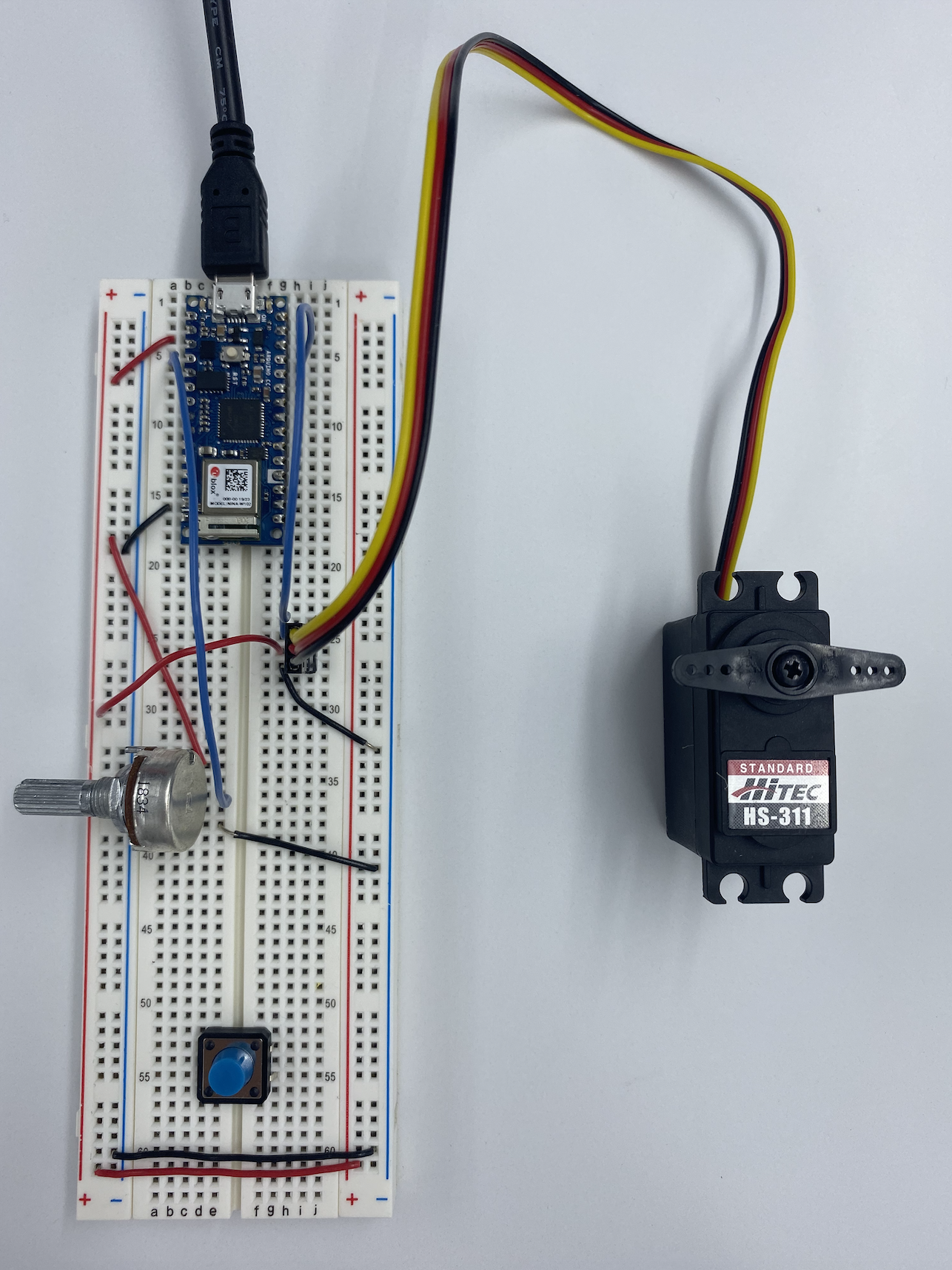
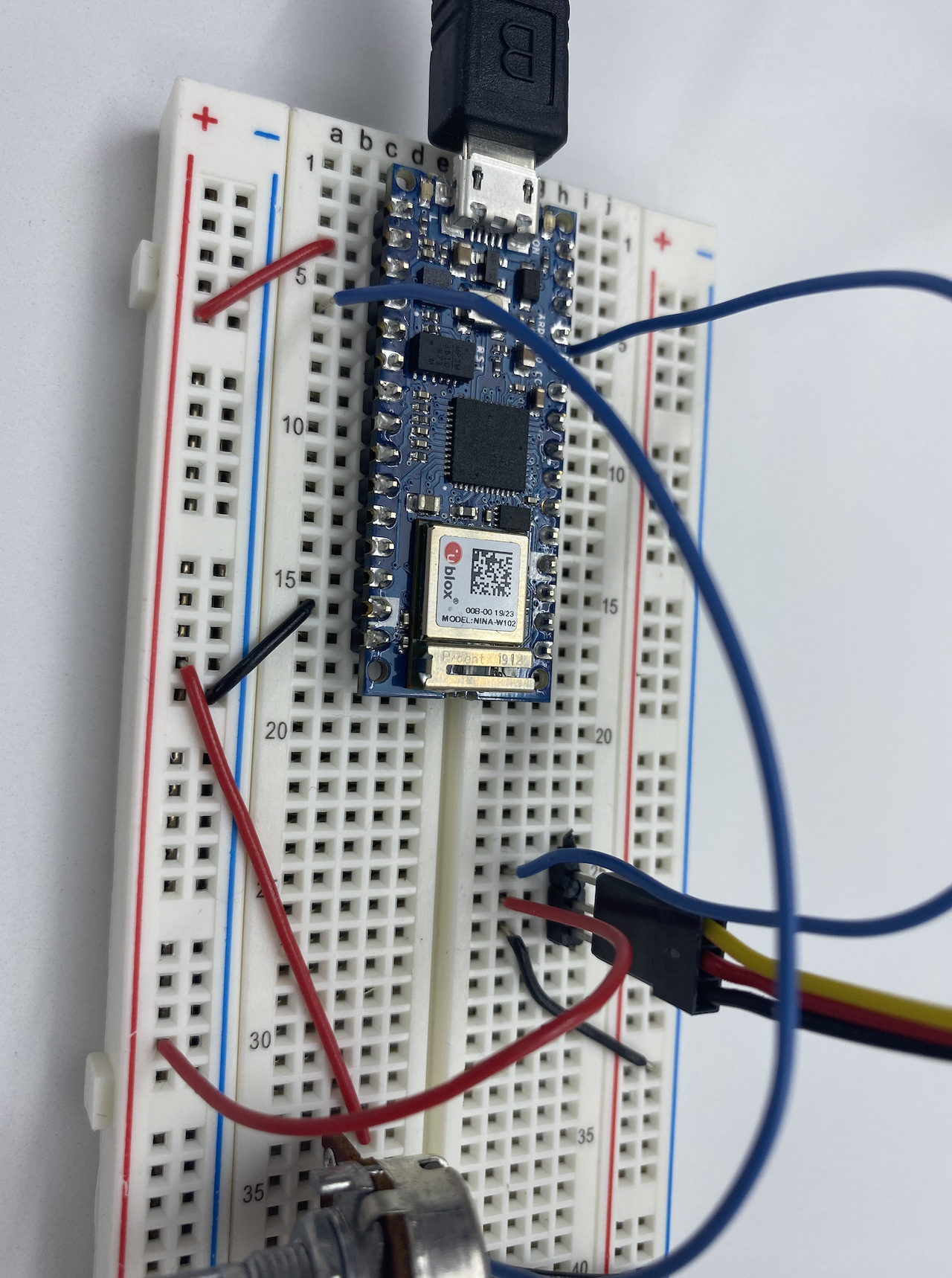
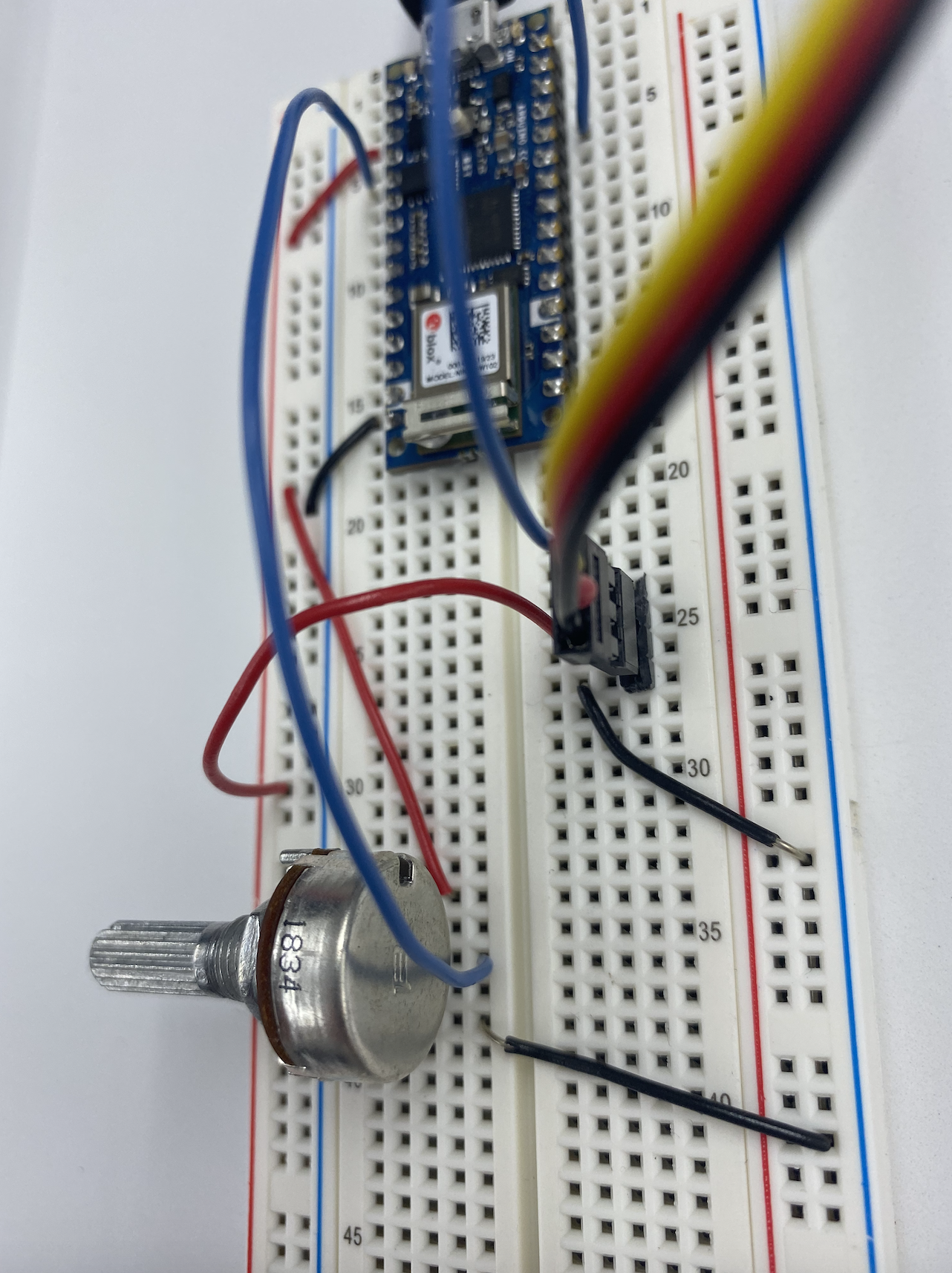
ANALOG - Servo Motor Control
//1. Find out range of sensor void setup() { Serial.begin(9600); // initialize serial communications } void loop() { int analogValue = analogRead(A0); // read the analog input Serial.println(analogValue); // print it }
//2.Map the result into 0-179 void setup() { Serial.begin(9600); // initialize serial communications } void loop() { int analogValue = analogRead(A0); // read the analog input Serial.println(analogValue); // print it int servoAngle = map(analogValue, 0, 1023, 0, 359); //}
So I had a questions on this step.
Where does 9600 come from: ‘Serial.begin(9600)’
Where does 1023 come from: ‘int servoAngle = map(analogValue, 0, 1023, 0, 179)’
//3. #include <Servo.h> // include servo library Servo servoMotor; int servoPin = 9; void setup() { Serial.begin(9600); servoMotor.attach(servoPin); } void loop() { int analogValue = analogRead(A0); Serial.println(analogValue); //0-1023 int servoAngle = map(analogValue,0,1023, 0,360); Serial.println(servoAngle); servoMotor.write(analogValue);
Creative Application
Idea sketch
INPUT/ OUTPUT
INPUT/ OUTPUT ARDUINO
I learned about INPUT and OUTPUT with Arduino.
So, I created this microcontroller circuit that has 1 DIGITAL INPUT and 2 OUTPUT
To start with, I connected power and ground on the breadboard to power and ground from the
microcontroller. Then I connected red and blue columns on the left of the breadboard
to the red and blue columns on the right side of the breadboard with red and black wires.
I wondered WHY? there should be a resistor after D2 wire.
What I learned?
The resistor connecting the pushbutton is a pulldown resistor.
It provides the digital input pin with a connection to ground. Without it, the input will behave unreliably.
Then I created additional 2 circuits connected to D3 and D4 which is going to be my output.
I connected resistors for both of them. Stronger resistor will make light bulbs dimmer.
Then I connected another side of lightbulb to negative(-).
I was curious why should it be connected to - and I found out each Arduino pin works as a +.
And I coded to light it up sequentially. By doing this, I could understand basic concept of how electric devices that interacts with people work.
At this point, I could not find out and other creative way to code and make creative circuit but I can’t wait to learn.
Now, I get the basic idea of INPUT and OUTPUT of Microcontroller.
<ARDUINO CODE>
void setup() { pinMode(2, INPUT); pinMode(3, OUTPUT); pinMode(4, OUTPUT); } void loop() { if (digitalRead(2) == HIGH) { digitalWrite(3, HIGH); digitalWrite(4, LOW); } else { digitalWrite(3, LOW); digitalWrite(4, HIGH); } }
OBSERVATION
Interactive technology I chose is an NYC metro card vending machine that I've been using for 7 years. People use it to refill their card; to get a new card, or to get a single ride card. People use it in many different ways. Some people choose their preferred language first; some people choose what they want first(get a new card, refill your card, single ride) and some people just insert the metro card right away so that the machine can skip previous steps and direct them to 'refill your card' screen right away.
Even though the machine is very straight forward,(does not have any other functions other than a metro card) elders or people who are new to New York City seems to have a hard time using it which causes a long line.
I used the machine for so long and have not thought about once what I liked about it. Probably, touch screen. The first user will never expect that such an old-looking machine is a touchscreen. It makes everything so much easier.
It takes the most time to Wait in Line to use the machine (Line created by tourists and first-time user). I guess the user interface of a metro vending machine needs some improvements. I think it would be easier and faster to use a chip, instead of the barcode(card). It takes only a few seconds for a machine to read the barcode but it feels long when there is a line. It can get to minutes or hours when seconds are added up.
NYC metro vending was an innovative technology once but not anymore.
For better user experience, it has to adopt new technology.
Train Your Bug
First I came up with really simple circuit that turns on the light.
Then I chose a part to add a switch.
My first idea was to use charcoal(pencil) as a conductor. I was going to make a maze with insulator and make user to figure out the maze in order to turn the light on. However, I found out that charcoal is not a great conductor. It turns the light on but not bright enough.
Then I found this cute looking ladybug toy I had. I connected wire to the front leg and covered it with aluminum foil. Then I connected those legs into single wire and +.
I wanted to keep the maze idea. So I made a track covered with conductor then connected it with - of the circuit. So that whenever machine bug touches the wall, the light will turn on.
While I was playing with this machine but, I found out that it is really hard to control. I thought it would be fun to make this experience as a simple game. ‘Train your machine Bug.’ Point of this designed circuit is to make you proficient at controlling your bug. User should try not to illuminate the lightbulb.
Also, you can adjust the difficulty by relocate the bar that makes a track. It’s a heavy metal covered with aluminum foil, so you can place it anywhere unless one side of it touches the wall.
NOW! Please enjoy ‘Train your machine bug’
The Art of Interactive Design
Chapter 1: What Exactly IS Interactivity?
- The term interactivity is overused and understood
- interaction: a cyclic process in which two actors alternately listen, think, and speak.
- listen, think, and speak = input, process, and output,
- ex/ branch, refrigerator à NOT an interactivity that has some blood in its veins.
- “high” and “low” interactivity?
- To interact well, both actors in a conversation must perform all three steps (listening, thinking, and speaking) well
- The most common design error in interactive products arises from a failure to appreciate this principle.
- A book can’t listen or think. It can only speak;
- Interaction and reaction are different.
- Participation and interaction are different.
- “If the movie were interactive, you might see our heroine pause and say, “Gee, I think I heard somebody in the audience urging me not to enter the dark house. I think I’ll take that advice.” But this never happens”
- “let’s be honest” – I think it is an interaction but it isn’t
- The user interface designer optimizes the design towards the computer’s strengths in speaking and listening, and away from its weaknesses in these same areas. The user interface designer never presumes to address the thinking content of software
- interactivity designer by regarding the thinking content of software as its function, and the user interface as its form
people tend define ‘interaction’ comprehensively. But interaction comes from something more fundamental. On the other hand, it is more than just fundamental.
I believe, ‘why’ is most important keyword in ‘interaction’
During my Thesis class at Parsons, professor kept asking me ‘why?’ to what I wanted to do.
Now I understand what he meant.
Ex/
I’ll make a graphic novel with AR technology so that I can animate the static book.
Why do you want to use AR technology?
Why would people use AR to read a book instead of watching some motion graphic?
‘Cool thing’, ‘Interesting effect’ is not really what interaction is about.
I think, to give an attribute of ‘interactivity,’ it has to have a purpose and reason for interaction.
1. Are rugs interactive? Explain in your own words why or why not.
No, because rug does not satisfy ‘thinking’ procedure.
2. Come up with your own damn definition of interactivity.
It’s an alternate constant action and reaction relationship between two things with a purpose.
Chapter 2: Why Bother With Interactivity?
- Interactivity is important for designers because it is a new and revolutionary communication medium
- For artists, interactivity represents an exciting and unexplored field of effort.
- most mammalian infants learn basic skills through interactive play
- interactivity: it engages the human mind more powerfully than any other form of expression: people identify more closely with it because they are emotionally right in the middle of it.
- “I hear and I forget; I see and I remember; I do and I understand.”
- Software designers who try to compete with movies, music, or printed graphics are guaranteed to lose. The one and only place where we can beat those other industries is in our interactivity
In a modern society, all sectors are organically intertwined with one another, sharing their inspirations, knowledge and technology.
I, as a designer, cherish myself as a contributor to accelerating a diverse range of human development through interactivity.
I realized that for more in-depth understanding of the world of media art, learning how to program and code is crucial as the Dean of Parsons Communication Design, Kang said, “The age of differentiating design and technology has ended”.
If computer is capable of doing what people can do. And if they can do better jobs (including programming). What makes human a human?
Fantasy Device
INPUT: Breathe
OUTPUT: Emotion Sticker & mint
Our group designed imaginary device that provides a sticker that shows emotion based on the data collected from users’ breathe.
Screen in the front will detect user’s numeric value of hormone and predict his/her emotion. (will also provide mint in order to make people involved)
This device can be used at the bar, party, conference etc.
"The things we make are less important than the relationships they support"
Tom Igoe
Physical computing
Building interactive physical systems
That can sense and respond to the analog world
- Dan & Toms Book
Interaction
A cyclic process in which two actors alternately listen, think, and speak
- Chris Crawford
Good interaction
Affordance
Intuitivity
Feedback
Involving Full body